- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries

How to Fix - UnboundLocalError: Local variable Referenced Before Assignment in Python
Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches.
What is UnboundLocalError: Local variable Referenced Before Assignment?
This error occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Below, are the reasons by which UnboundLocalError: Local variable Referenced Before Assignment error occurs in Python :
Nested Function Variable Access
Global variable modification.
In this code, the outer_function defines a variable 'x' and a nested inner_function attempts to access it, but encounters an UnboundLocalError due to a local 'x' being defined later in the inner_function.
In this code, the function example_function tries to increment the global variable 'x', but encounters an UnboundLocalError since it's treated as a local variable due to the assignment operation within the function.
Solution for Local variable Referenced Before Assignment in Python
Below, are the approaches to solve “Local variable Referenced Before Assignment”.
In this code, example_function successfully modifies the global variable 'x' by declaring it as global within the function, incrementing its value by 1, and then printing the updated value.
In this code, the outer_function defines a local variable 'x', and the inner_function accesses and modifies it as a nonlocal variable, allowing changes to the outer function's scope from within the inner function.
Similar Reads
- Python Programs
- Python Errors
- Python How-to-fix
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Fixing Python UnboundLocalError: Local Variable ‘x’ Accessed Before Assignment
Understanding unboundlocalerror.
The UnboundLocalError in Python occurs when a function tries to access a local variable before it has been assigned a value. Variables in Python have scope that defines their level of visibility throughout the code: global scope, local scope, and nonlocal (in nested functions) scope. This error typically surfaces when using a variable that has not been initialized in the current function’s scope or when an attempt is made to modify a global variable without proper declaration.
Solutions for the Problem
To fix an UnboundLocalError, you need to identify the scope of the problematic variable and ensure it is correctly used within that scope.
Method 1: Initializing the Variable
Make sure to initialize the variable within the function before using it. This is often the simplest fix.
Method 2: Using Global Variables
If you intend to use a global variable and modify its value within a function, you must declare it as global before you use it.
Method 3: Using Nonlocal Variables
If the variable is defined in an outer function and you want to modify it within a nested function, use the nonlocal keyword.
That’s it. Happy coding!
Next Article: Fixing Python TypeError: Descriptor 'lower' for 'str' Objects Doesn't Apply to 'dict' Object
Previous Article: Python TypeError: write() argument must be str, not bytes
Series: Common Errors in Python and How to Fix Them
Related Articles
- Python Warning: Secure coding is not enabled for restorable state
- 4 ways to install Python modules on Windows without admin rights
- Python TypeError: object of type ‘NoneType’ has no len()
- Python: How to access command-line arguments (3 approaches)
- Understanding ‘Never’ type in Python 3.11+ (5 examples)
- Python: 3 Ways to Retrieve City/Country from IP Address
- Using Type Aliases in Python: A Practical Guide (with Examples)
- Python: Defining distinct types using NewType class
- Using Optional Type in Python (explained with examples)
- Python: How to Override Methods in Classes
- Python: Define Generic Types for Lists of Nested Dictionaries
- Python: Defining type for a list that can contain both numbers and strings
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js

Top 2 Methods to Solve the ‘Local Variable Referenced Before Assignment’ Error in Python
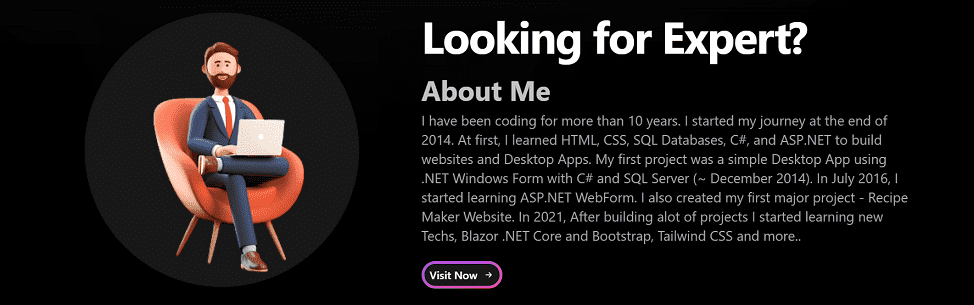
Table of Contents
When working with Python, encountering the UnboundLocalError can be quite common, especially when dealing with variables that you intend to access globally within a function. This error typically occurs when a variable is referenced before it has been assigned a value within the local scope.
The Problem: Local Variable Referenced Before Assignment
Consider the following example:
Running the code above yields the error:
The critical point here is whether the variable test1 is recognized as global or local. In this case, Python reinterprets test1 as a local variable due to the attempted modification with += , which leads to confusion when it’s referenced before being assigned any value in the local scope.
So how can you resolve this issue effectively without passing test1 as an argument into test_func ? Let’s explore two main methods to approach this.
Method 1: Avoiding Globals
The best practice suggests minimizing the use of global variables. Instead of modifying a global variable directly, consider passing the variable to a function. Here’s how you could rewrite the example to avoid using a global variable entirely:
In this example, test_func takes a parameter x , performs the operation, and returns the modified value, allowing us to keep the variable scope clean.
Method 2: Declaring a Variable as Global
If modifying a global variable within a function is necessary, use the global keyword. Here’s how you can clarify that test1 should be treated as a global variable within test_func :
By using global test1 , you inform Python of your intention to operate on the global instance of test1 , thus eliminating the UnboundLocalError .
Further Alternatives
While the two methods outlined above are the most straightforward solutions, you can also consider using classes to encapsulate your variables and methods, managing state more formally through object-oriented programming. Here’s a simple example:
This alternative approach provides a structured way to manage your variables, improving code readability and maintainability.

FAQs on Top 2 Methods to Solve the ‘Local Variable Referenced Before Assignment’ Error in Python
Q: what is unboundlocalerror in python, q: how can i avoid using global variables in python, q: does using the global keyword affect performance, q: what are the best practices for variable scope in python.
For additional resources on Python programming, you might find W3Schools Python Tutorials and Geeks for Geeks Python Programming useful.
[SOLVED] Local Variable Referenced Before Assignment
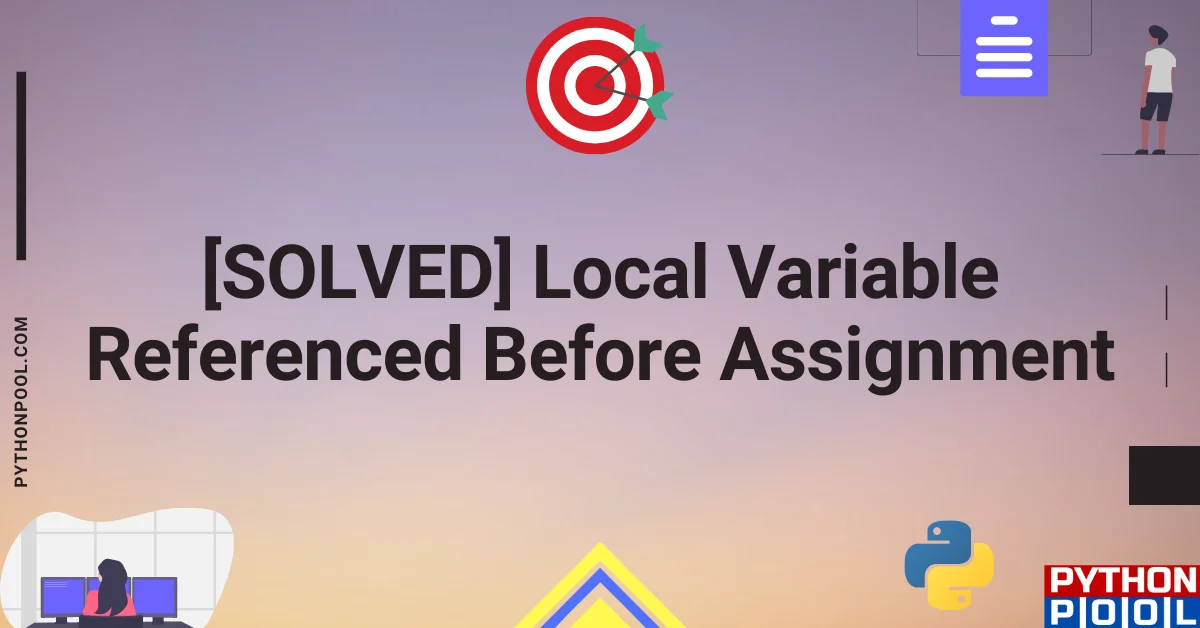
Python treats variables referenced only inside a function as global variables. Any variable assigned to a function’s body is assumed to be a local variable unless explicitly declared as global.
Why Does This Error Occur?
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Hence it searches for the variable whenever used. When not found, it throws the error.
Before we hop into the solutions, let’s have a look at what is the global and local variables.
Local Variable Declarations vs. Global Variable Declarations
![unboundlocalerror local variable 'range' referenced before assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
Local Variable Referenced Before Assignment Error with Explanation
Try these examples yourself using our Online Compiler.
Let’s look at the following function:

Explanation
The variable myVar has been assigned a value twice. Once before the declaration of myFunction and within myFunction itself.
Using Global Variables
Passing the variable as global allows the function to recognize the variable outside the function.
Create Functions that Take in Parameters
Instead of initializing myVar as a global or local variable, it can be passed to the function as a parameter. This removes the need to create a variable in memory.
UnboundLocalError: local variable ‘DISTRO_NAME’
This error may occur when trying to launch the Anaconda Navigator in Linux Systems.
Upon launching Anaconda Navigator, the opening screen freezes and doesn’t proceed to load.
Try and update your Anaconda Navigator with the following command.
If solution one doesn’t work, you have to edit a file located at
After finding and opening the Python file, make the following changes:
In the function on line 159, simply add the line:
DISTRO_NAME = None
Save the file and re-launch Anaconda Navigator.
DJANGO – Local Variable Referenced Before Assignment [Form]
The program takes information from a form filled out by a user. Accordingly, an email is sent using the information.
Upon running you get the following error:
We have created a class myForm that creates instances of Django forms. It extracts the user’s name, email, and message to be sent.
A function GetContact is created to use the information from the Django form and produce an email. It takes one request parameter. Prior to sending the email, the function verifies the validity of the form. Upon True , .get() function is passed to fetch the name, email, and message. Finally, the email sent via the send_mail function
Why does the error occur?
We are initializing form under the if request.method == “POST” condition statement. Using the GET request, our variable form doesn’t get defined.
Local variable Referenced before assignment but it is global
This is a common error that happens when we don’t provide a value to a variable and reference it. This can happen with local variables. Global variables can’t be assigned.
This error message is raised when a variable is referenced before it has been assigned a value within the local scope of a function, even though it is a global variable.
Here’s an example to help illustrate the problem:
In this example, x is a global variable that is defined outside of the function my_func(). However, when we try to print the value of x inside the function, we get a UnboundLocalError with the message “local variable ‘x’ referenced before assignment”.
This is because the += operator implicitly creates a local variable within the function’s scope, which shadows the global variable of the same name. Since we’re trying to access the value of x before it’s been assigned a value within the local scope, the interpreter raises an error.
To fix this, you can use the global keyword to explicitly refer to the global variable within the function’s scope:
However, in the above example, the global keyword tells Python that we want to modify the value of the global variable x, rather than creating a new local variable. This allows us to access and modify the global variable within the function’s scope, without causing any errors.
Local variable ‘version’ referenced before assignment ubuntu-drivers
This error occurs with Ubuntu version drivers. To solve this error, you can re-specify the version information and give a split as 2 –
Here, p_name means package name.
With the help of the threading module, you can avoid using global variables in multi-threading. Make sure you lock and release your threads correctly to avoid the race condition.
When a variable that is created locally is called before assigning, it results in Unbound Local Error in Python. The interpreter can’t track the variable.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
Trending Python Articles
![unboundlocalerror local variable 'range' referenced before assignment [Fixed] nameerror: name Unicode is not defined](https://www.pythonpool.com/wp-content/uploads/2024/01/Fixed-nameerror-name-Unicode-is-not-defined-300x157.webp)
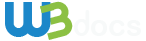
- Password Generator
- HTML Editor
- HTML Encoder
- JSON Beautifier
- CSS Beautifier
- Markdown Convertor
- Find the Closest Tailwind CSS Color
- Phrase encrypt / decrypt
- Browser Feature Detection
- Number convertor
- CSS Maker text shadow
- CSS Maker Text Rotation
- CSS Maker Out Line
- CSS Maker RGB Shadow
- CSS Maker Transform
- CSS Maker Font Face
- Color Picker
- Colors CMYK
- Color mixer
- Color Converter
- Color Contrast Analyzer
- Color Gradient
- String Length Calculator
- MD5 Hash Generator
- Sha256 Hash Generator
- String Reverse
- URL Encoder
- URL Decoder
- Base 64 Encoder
- Base 64 Decoder
- Extra Spaces Remover
- String to Lowercase
- String to Uppercase
- Word Count Calculator
- Empty Lines Remover
- HTML Tags Remover
- Binary to Hex
- Hex to Binary
- Rot13 Transform on a String
- String to Binary
- Duplicate Lines Remover
Python 3: UnboundLocalError: local variable referenced before assignment
This error occurs when you are trying to access a variable before it has been assigned a value. Here is an example of a code snippet that would raise this error:
Watch a video course Python - The Practical Guide
The error message will be:
In this example, the variable x is being accessed before it is assigned a value, which is causing the error. To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement.
Both will work without any error.
Related Resources
- Using global variables in a function
- "Least Astonishment" and the Mutable Default Argument
- Why is "1000000000000000 in range(1000000000000001)" so fast in Python 3?
- HTML Basics
- Javascript Basics
- TypeScript Basics
- React Basics
- Angular Basics
- Sass Basics
- Vue.js Basics
- Python Basics
- Java Basics
- NodeJS Basics
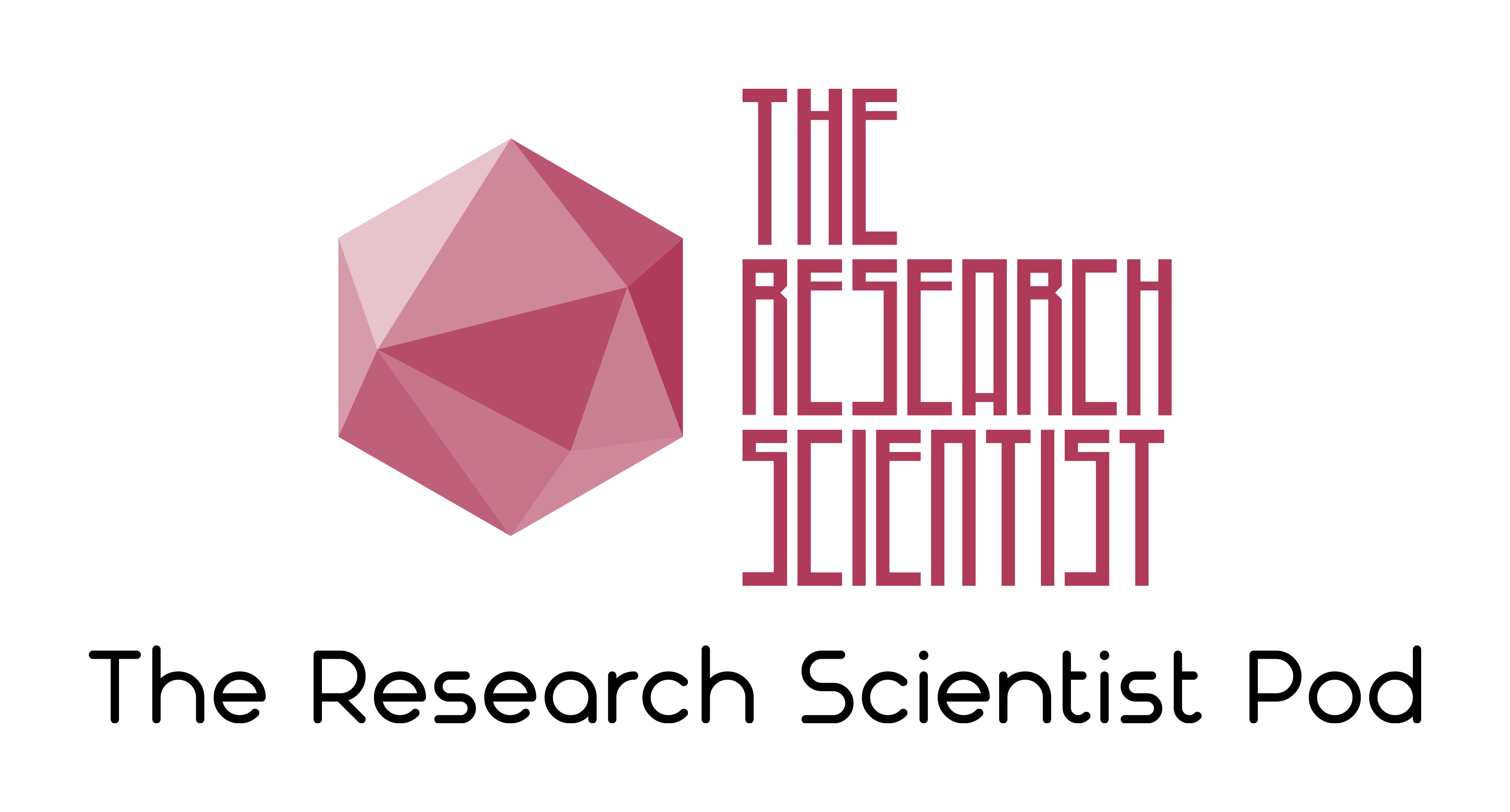
Python UnboundLocalError: local variable referenced before assignment
by Suf | Programming , Python , Tips
If you try to reference a local variable before assigning a value to it within the body of a function, you will encounter the UnboundLocalError: local variable referenced before assignment.
The preferable way to solve this error is to pass parameters to your function, for example:
Alternatively, you can declare the variable as global to access it while inside a function. For example,
This tutorial will go through the error in detail and how to solve it with code examples .
Table of contents
What is scope in python, unboundlocalerror: local variable referenced before assignment, solution #1: passing parameters to the function, solution #2: use global keyword, solution #1: include else statement, solution #2: use global keyword.
Scope refers to a variable being only available inside the region where it was created. A variable created inside a function belongs to the local scope of that function, and we can only use that variable inside that function.
A variable created in the main body of the Python code is a global variable and belongs to the global scope. Global variables are available within any scope, global and local.
UnboundLocalError occurs when we try to modify a variable defined as local before creating it. If we only need to read a variable within a function, we can do so without using the global keyword. Consider the following example that demonstrates a variable var created with global scope and accessed from test_func :
If we try to assign a value to var within test_func , the Python interpreter will raise the UnboundLocalError:
This error occurs because when we make an assignment to a variable in a scope, that variable becomes local to that scope and overrides any variable with the same name in the global or outer scope.
var +=1 is similar to var = var + 1 , therefore the Python interpreter should first read var , perform the addition and assign the value back to var .
var is a variable local to test_func , so the variable is read or referenced before we have assigned it. As a result, the Python interpreter raises the UnboundLocalError.
Example #1: Accessing a Local Variable
Let’s look at an example where we define a global variable number. We will use the increment_func to increase the numerical value of number by 1.
Let’s run the code to see what happens:
The error occurs because we tried to read a local variable before assigning a value to it.
We can solve this error by passing a parameter to increment_func . This solution is the preferred approach. Typically Python developers avoid declaring global variables unless they are necessary. Let’s look at the revised code:
We have assigned a value to number and passed it to the increment_func , which will resolve the UnboundLocalError. Let’s run the code to see the result:
We successfully printed the value to the console.
We also can solve this error by using the global keyword. The global statement tells the Python interpreter that inside increment_func , the variable number is a global variable even if we assign to it in increment_func . Let’s look at the revised code:
Let’s run the code to see the result:
Example #2: Function with if-elif statements
Let’s look at an example where we collect a score from a player of a game to rank their level of expertise. The variable we will use is called score and the calculate_level function takes in score as a parameter and returns a string containing the player’s level .
In the above code, we have a series of if-elif statements for assigning a string to the level variable. Let’s run the code to see what happens:
The error occurs because we input a score equal to 40 . The conditional statements in the function do not account for a value below 55 , therefore when we call the calculate_level function, Python will attempt to return level without any value assigned to it.
We can solve this error by completing the set of conditions with an else statement. The else statement will provide an assignment to level for all scores lower than 55 . Let’s look at the revised code:
In the above code, all scores below 55 are given the beginner level. Let’s run the code to see what happens:
We can also create a global variable level and then use the global keyword inside calculate_level . Using the global keyword will ensure that the variable is available in the local scope of the calculate_level function. Let’s look at the revised code.
In the above code, we put the global statement inside the function and at the beginning. Note that the “default” value of level is beginner and we do not include the else statement in the function. Let’s run the code to see the result:
Congratulations on reading to the end of this tutorial! The UnboundLocalError: local variable referenced before assignment occurs when you try to reference a local variable before assigning a value to it. Preferably, you can solve this error by passing parameters to your function. Alternatively, you can use the global keyword.
If you have if-elif statements in your code where you assign a value to a local variable and do not account for all outcomes, you may encounter this error. In which case, you must include an else statement to account for the missing outcome.
For further reading on Python code blocks and structure, go to the article: How to Solve Python IndentationError: unindent does not match any outer indentation level .
Go to the online courses page on Python to learn more about Python for data science and machine learning.
Have fun and happy researching!
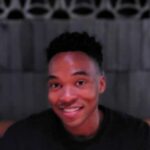
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.
- Suf https://researchdatapod.com/author/soofyserial/ How to Solve Python ModuleNotFoundError: no module named ‘thread’
- Suf https://researchdatapod.com/author/soofyserial/ How to Solve Python TypeError: 'builtin_function_or_method' object is not iterable
- Suf https://researchdatapod.com/author/soofyserial/ How to Convert String to Char Array in C++
- Suf https://researchdatapod.com/author/soofyserial/ How to Add an Index column to a Data Frame in R
Buy Me a Coffee

IMAGES
VIDEO
COMMENTS
This is because, even though Var1 exists, you're also using an assignment statement on the name Var1 inside of the function (Var1 -= 1 at the bottom line). Naturally, this creates a variable inside the function's scope called Var1 (truthfully, a -= or += will only update (reassign) an existing variable, but for reasons unknown (likely consistency in this context), Python treats it as an ...
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
Method 1: Initializing the Variable. Make sure to initialize the variable within the function before using it. This is often the simplest fix. Method 2: Using Global Variables. If you intend to use a global variable and modify its value within a function, you must declare it as global before you use it. Method 3: Using Nonlocal Variables
UnboundLocalError: local variable 'test1' referenced before assignment. The critical point here is whether the variable test1 is recognized as global or local. In this case, Python reinterprets test1 as a local variable due to the attempted modification with += , which leads to confusion when it's referenced before being assigned any value in ...
0. Your error: UnboundLocalError: local variable 'list1' referenced before assignment was coming because you have not defined list1 in medianOfList (self) but you are trying to access it. Here's my solution: def __init__(self,list1,list2): self.list1=list1. self.list2=list2. def medianOfList(self,list1,list2):
The row variable is initialized by the inner for loop, but is then accessed outside. Quoting the official documentation:. The for-loop makes assignments to the variables(s) in the target list. [...] Names in the target list are not deleted when the loop is finished, but if the sequence is empty, they will not have been assigned to at all by the loop.
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Python does not have the concept of variable declarations.
To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement. def example (): x = 5 print (x) example()
UnboundLocalError: local variable referenced before assignment. Example #1: Accessing a Local Variable. Solution #1: Passing Parameters to the Function. Solution #2: Use Global Keyword. Example #2: Function with if-elif statements. Solution #1: Include else statement. Solution #2: Use global keyword. Summary.
You can't assign a new variable in an if/elif block without an else block that catches all the cases your if/elifs don't. You can fix this with an else block, or by assigning range some default value before the if.