- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions

Assignment Operators in C
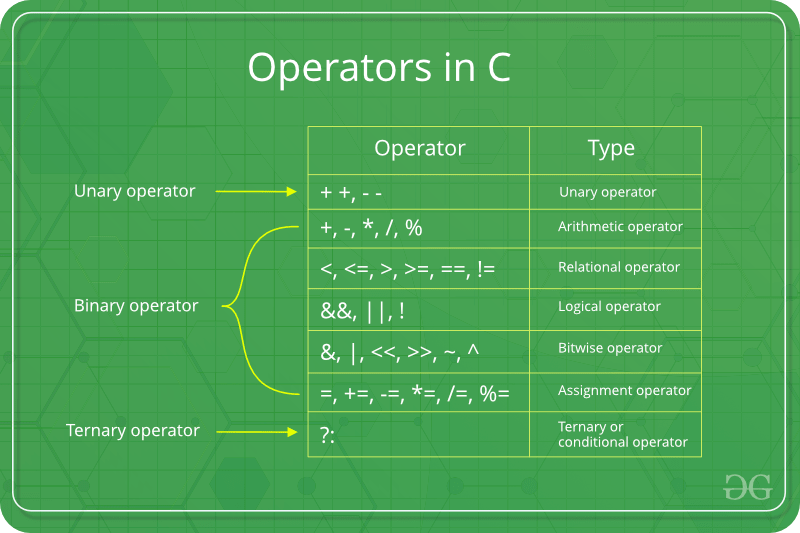
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Similar Reads
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Explain the variable declaration, initialization and assignment in C language
The main purpose of variables is to store data in memory. Unlike constants, it will not change during the program execution. However, its value may be changed during execution.
The variable declaration indicates that the operating system is going to reserve a piece of memory with that variable name.

Variable declaration
The syntax for variable declaration is as follows −
For example,
Here, a, b, c, d are variables. The int, float, double are the data types.
Variable initialization
The syntax for variable initialization is as follows −
Variable Assignment
A variable assignment is a process of assigning a value to a variable.
Rules for defining variables
A variable may be alphabets, digits, and underscore.
A variable name can start with an alphabet, and an underscore but, can’t start with a digit.
Whitespace is not allowed in the variable name.
A variable name is not a reserved word or keyword. For example, int, goto etc.
Following is the C program for variable assignment −
Live Demo
When the above program is executed, it produces the following result −

- Related Articles
- Initialization, declaration and assignment terms in Java
- Explain variable declaration and rules of variables in C language
- Variable initialization in C++
- What is the difference between initialization and assignment of values in C#?
- Structure declaration in C language
- Explain the accessing of structure variable in C language
- Explain scope of a variable in C language.
- Explain Lifetime of a variable in C language.
- Explain Binding of a variable in C language.
- Initialization of variable sized arrays in C
- MySQL temporary variable assignment?
- Explain Compile time and Run time initialization in C programming?
- Rules For Variable Declaration in Java
- Explain the Difference Between Definition and Declaration
- Java variable declaration best practices
Kickstart Your Career
Get certified by completing the course

IMAGES
VIDEO